In this project, we will learn how to adjust the brightness of an LED using an Arduino Uno board.
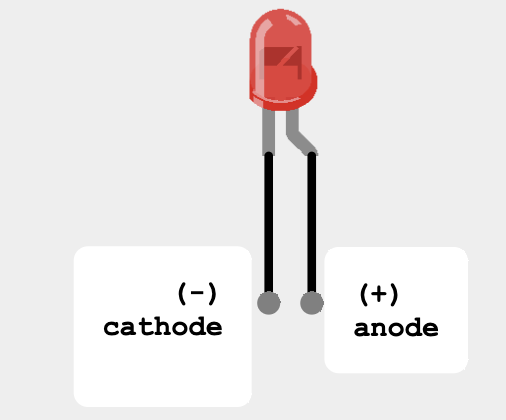
LED
An LED glows when the voltage at the anode becomes positive relative to the voltage at the cathode.
Brightness Control
The brightness of an led depends on
- Current through it.
- Forward voltage across it.
In digital control, the brightness can be controlled using PWM. By rapidly switching the LED on and off at a high frequency, the average current and hence the brightness can be adjusted. The duty cycle of the PWM signal determines the brightness.
Circuit Wiring
Program Code
/* www.matthewtechub.com
About program…
Automatically increase the brightness of led.
Then turn off and process repeated
*/
// initialise LED pin
const int ledPin = 8;
// Variable to store the brightness level
int brightness = 0;
void setup() {
// Set ledPin as an output
pinMode(ledPin, OUTPUT);
}
void loop() {
// Set the brightness of the LED
analogWrite(ledPin, brightness);
// Change the brightness for next time through the loop
brightness = brightness + 5;
// Check whether maximum brightness reached.
if (brightness >= 255)
{
// Dim LED.
brightness=0;
}
// Wait for 30 milliseconds to see the dimming effect
delay(50);
}
Code Explanation
const int ledPin = 8;
- Declares a constant integer variable named
ledPin
and initializes it with the value 8, signifying that the LED is connected to pin 8. - Using
const
ensures that the pin number cannot be accidentally changed later in the code, which helps prevent errors.
int brightness = 0;
Set initial brightness 0
pinMode(ledPin, OUTPUT);
Configures the specified pin (ledPin
, which is pin 8 in this case) as an output. This allows the pin to send signals, such as turning an LED on or off.
analogWrite(ledPin, brightness);
Used to control the brightness of an LED (or any other PWM-compatible device) by sending a Pulse Width Modulation (PWM) signal to a specified pin (pin 8 here) on the Arduino.
if (brightness >=
255)
Checks if the brightness value has reached or exceeded 255, which is the maximum brightness level for the LED.
Modify Yourself
- Checks if the brightness value has reached or exceeded 255, which is the maximum brightness level for the LED.
- Please modify the program to increase the brightness and then decrease it repeatedly.