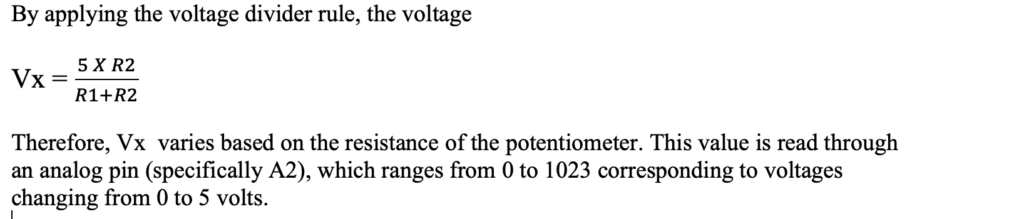
Program Code
// www.matthewtechub.com
// voltmeter(0-5 V)
int analogPin=A2;
int value;
float voltage;
void setup() {
// put your setup code here, to run once:
pinMode(analogPin,INPUT);
Serial.begin(9600);
}
void loop() {
// put your main code here, to run repeatedly:
value=analogRead(analogPin);
voltage= (float)value*5/1024;
delay(500);
Serial.print("Voltage= ");
Serial.println(voltage);
}
Code Explanation
int analogPin = A2; // Pin connected to the potentiometer (analog input pin A2)
int value; // Variable to store the analog reading
float voltage; // Variable to store the calculated voltage
- analogPin: Defines the Arduino pin number (A2) to which the potentiometer is connected.
- value: Integer variable used to store the analog reading obtained from the potentiometer.
- voltage: Float variable used to store the calculated voltage based on the analog reading.
void setup() {
pinMode(analogPin, INPUT); // Configures analogPin (A2) as an input pin to read analog data
Serial.begin(9600); // Initializes serial communication with baud rate 9600
}
- pinMode(analogPin, INPUT);: Configures analogPin (A2) as an input pin, indicating it will read analog data from the potentiometer.
- Serial.begin(9600);: Initializes serial communication between Arduino and the connected computer at a baud rate of 9600 bits per second. This allows data to be sent and received via the Serial Monitor.
void loop() {
value = analogRead(analogPin); // Reads the analog value from analogPin (A2) and stores it in ‘value’
voltage = (float)value * 5 / 1024; // Calculates the voltage based on the analog reading
delay(500); // Delays for 500 milliseconds (0.5 seconds) for stability
Serial.print(“Voltage= “); // Prints the label “Voltage= ” to the Serial Monitor
Serial.println(voltage); // Prints the calculated voltage to the Serial Monitor
}
- analogRead(analogPin);: Reads the analog voltage value from the potentiometer connected to analogPin (A2) and assigns it to the variable value.
- Voltage Calculation:
- voltage = (float)value * 5 / 1024;: Converts the analog reading (value) into a voltage value. The Arduino’s analog-to-digital converter (ADC) provides values from 0 to 1023 for voltages from 0 to 5 volts.
- Serial Output:
- Serial.print(“Voltage= “);: Prints the label “Voltage= ” to the Serial Monitor.
- Serial.println(voltage);: Prints the calculated voltage (stored in voltage) to the Serial Monitor followed by a newline character (\n).