About The Project
In this project, we will learn how to increment a counter each time the touch sensor is activated and print the count to the Serial Monitor.
Touch Sensor
The TTP223-1 is a capacitive touch sensor module used to detect touch input. When touched, its output pin goes HIGH; otherwise, the output remains LOW.
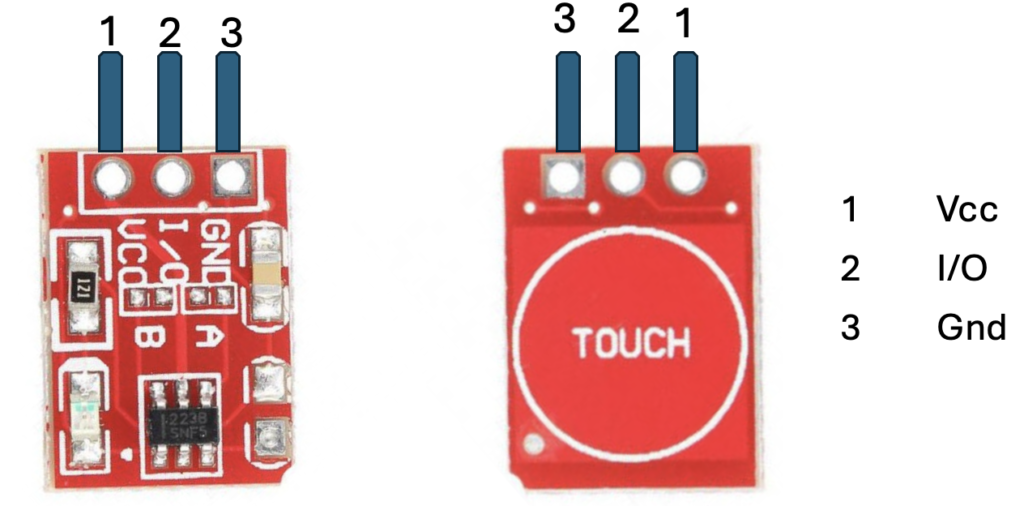
Circuit Wiring
Program Code
C++
// www.matthewtechub.com
// counting number of touches
const int touchPin = 2;
// Pin connected to the touch sensor module
int touchCount = 0;
// Variable to count the number of touches
int lastTouchState = LOW;
// Variable to store the last state of the touch sensor
void setup()
{
Serial.begin(9600);
// Initialize serial communication at 9600 baud rate
pinMode(touchPin, INPUT);
// Set the touch pin as an input
}
void loop()
{
int touchState = digitalRead(touchPin);
// Read the current state of the touch sensor
// Check if the touch sensor state has changed from LOW to HIGH
if (touchState == HIGH && lastTouchState == LOW) {
touchCount++;
// Increment the touch count
Serial.print("Touch count: ");
Serial.println(touchCount);
// Print the touch count to the Serial Monitor
}
// Update the last touch state
lastTouchState = touchState;
delay(100);
// Small delay to debounce the sensor
}
Code Explanation
C++
const int touchPin = 2;
// Pin connected to the touch sensor module
int touchCount = 0;
// Variable to count the number of touches
int lastTouchState = LOW;
// Variable to store the last state of the touch sensor
- touchPin: Specifies that the touch sensor is connected to digital pin 2 of the Arduino.
- touchCount: An integer variable to keep track of the number of touches detected.
- lastTouchState: An integer variable to store the previous state of the touch sensor, initialized to LOW.
C++
void setup()
{
Serial.begin(9600);
// Initialize serial communication at 9600 baud rate
pinMode(touchPin, INPUT);
// Set the touch pin as an input
}
- Serial.begin(9600);: Initializes serial communication at a baud rate of 9600 bits per second, allowing the Arduino to communicate with the Serial Monitor.
- pinMode(touchPin, INPUT);: Configures the touchPin as an input pin to read the state of the touch sensor.
C++
void loop()
{
int touchState = digitalRead(touchPin);
// Read the current state of the touch sensor
- digitalRead(touchPin);: Reads the current state of the touch sensor pin. If the sensor is touched, it returns HIGH; otherwise, it returns LOW.
C++
// Check if the touch sensor state has changed from LOW to HIGH
if (touchState == HIGH && lastTouchState == LOW) {
touchCount++;
// Increment the touch count
Serial.print("Touch count: ");
Serial.println(touchCount);
// Print the touch count to the Serial Monitor
}
- The if statement checks if the touch sensor state has changed from LOW to HIGH, indicating a touch event.
- If a touch is detected, touchCount++ increments the touch count.
- Serial.print(“Touch count: “); and Serial.println(touchCount); print the updated touch count to the Serial Monitor.
C++
// Update the last touch state
lastTouchState = touchState;
delay(100);
// Small delay to debounce the sensor
}
- lastTouchState = touchState;: Updates the last touch state to the current state to detect changes in the next loop iteration.
- delay(100);: Adds a 100-millisecond delay to debounce the sensor and prevent multiple counts from a single touch.
Try Yourself
Modify the program code to count up to 10 with each touch. When the count reaches 10, it should count down to 0.