About The Project
In this project, we will create a pattern lock system using touch sensors, an LED, and a buzzer. The goal is to input a sequence of touches (a pattern) and compare it with a predefined password stored in the program. The system will provide feedback through an LED and a buzzer to indicate whether the entered pattern is correct or incorrect. The results will also be displayed on the Serial Monitor.
Touch Sensor
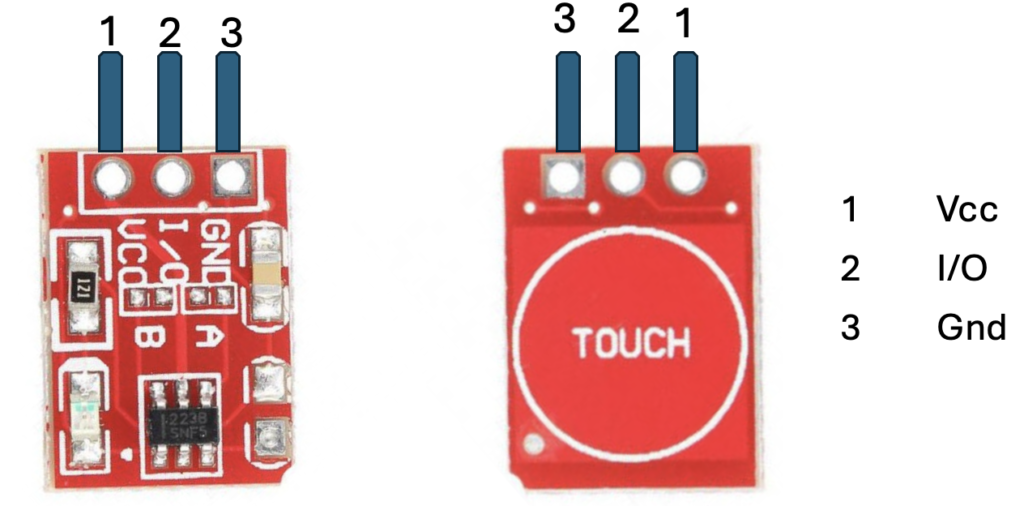
The TTP223-1 is a capacitive touch sensor module used to detect touch input. When touched, its output pin goes HIGH; otherwise, the output remains LOW.
Circuit Wiring
The original password is stored as an array in the program. First, press the button switch, then enter the 3-digit password by touching the appropriate touch sensors. If the entered password matches the original, the LED will turn on for 5 seconds. Otherwise, the buzzer will beep for 5 seconds. You can reenter the password by pressing the button switch again. The response can be monitored using the serial monitor.
Program Code
// www.matthewtechub.com
// Touch sensor password with LED and buzzer
const int touchPins[] = {2, 3, 4}; // Pins connected to the touch sensors
const int buttonPin = 5; // Pin connected to the button switch
const int ledPin = 6; // Pin connected to the LED
const int buzzerPin = 7; // Pin connected to the buzzer
const int password[] = {3, 1, 2}; // The password sequence (touch sensor 3, then 1, then 2)
const int passwordLength = 3; // Length of the password
int inputSequence[passwordLength]; // Array to store the input sequence
int inputIndex = 0; // Index to track input sequence
void setup() {
Serial.begin(9600); // Initialize serial communication
for (int i = 0; i < 3; i++) {
pinMode(touchPins[i], INPUT); // Set touch pins as inputs
}
pinMode(buttonPin, INPUT_PULLUP); // Set button pin as input with internal pull-up resistor
pinMode(ledPin, OUTPUT); // Set LED pin as output
pinMode(buzzerPin, OUTPUT); // Set buzzer pin as output
digitalWrite(ledPin, LOW); // Ensure LED is initially off
digitalWrite(buzzerPin, LOW); // Ensure buzzer is initially off
}
void loop() {
if (digitalRead(buttonPin) == LOW) { // Check if the button is pressed
delay(50); // Debounce delay
while (digitalRead(buttonPin) == LOW); // Wait for button release
inputIndex = 0; // Reset input sequence index
Serial.println("Enter password sequence:");
// Wait for password input
while (inputIndex < passwordLength) {
for (int i = 0; i < 3; i++) {
if (digitalRead(touchPins[i]) == HIGH) {
inputSequence[inputIndex] = i + 1; // Store the touch sensor number (1, 2, 3)
inputIndex++;
Serial.print("Touch sensor ");
Serial.println(i + 1);
delay(500); // Debounce delay
while (digitalRead(touchPins[i]) == HIGH); // Wait for sensor release
}
}
}
// Check if the entered sequence matches the password
bool isCorrect = true;
for (int i = 0; i < passwordLength; i++) {
if (inputSequence[i] != password[i]) {
isCorrect = false;
break;
}
}
if (isCorrect) {
digitalWrite(ledPin, HIGH); // Turn on the LED if password is correct
Serial.println("Password correct!");
} else {
digitalWrite(buzzerPin, HIGH); // Turn on the buzzer if password is incorrect
Serial.println("Password incorrect!");
delay(5000); // Buzzer on for 1 second
digitalWrite(buzzerPin, LOW);
}
delay(5000); // Wait 3 seconds before resetting
digitalWrite(ledPin, LOW); // Turn off the LED
Serial.println("Ready for new input.");
}
}
Code Explanation
// www.matthewtechub.com
// Touch sensor password with LED and buzzer
const int touchPins[] = {2, 3, 4};
// Pins connected to the touch sensors
const int buttonPin = 5;
// Pin connected to the button switch
const int ledPin = 6;
// Pin connected to the LED
const int buzzerPin = 7;
// Pin connected to the buzzer
const int password[] = {3, 1, 2};
// The password sequence (touch sensor 3, then 1, then 2)
const int passwordLength = 3;
// Length of the password
int inputSequence[passwordLength];
// Array to store the input sequence
int inputIndex = 0;
// Index to track input sequence
Declarations
- touchPins[ ]: Array of integers specifying the pin numbers connected to the touch sensors.
- buttonPin: Integer specifying the pin number connected to the button switch.
- ledPin: Integer specifying the pin number connected to the LED.
- buzzerPin: Integer specifying the pin number connected to the buzzer.
- password[ ]: Array storing the correct sequence of touch sensor activations (3, 1, 2).
- passwordLength: Integer specifying the length of the password.
- inputSequence[ ]: Array to store the user’s input sequence.
- inputIndex: Index to track the current position in the input sequence.
void setup() {
Serial.begin(9600); // Initialize serial communication
for (int i = 0; i < 3; i++) {
pinMode(touchPins[i], INPUT);
// Set touch pins as inputs
}
pinMode(buttonPin, INPUT_PULLUP); /
/ Set button pin as input with internal pull-up resistor
pinMode(ledPin, OUTPUT);
// Set LED pin as output
pinMode(buzzerPin, OUTPUT);
// Set buzzer pin as output
digitalWrite(ledPin, LOW);
// Ensure LED is initially off
digitalWrite(buzzerPin, LOW);
// Ensure buzzer is initially off
}
Setup Function
- Initializes serial communication for debugging purposes.
- Sets each touch sensor pin as an input.
- Sets the button pin as an input with an internal pull-up resistor (so it reads HIGH when not pressed).
- Sets the LED and buzzer pins as outputs.
- Ensures that the LED and buzzer are initially turned off.
void loop() {
if (digitalRead(buttonPin) == LOW) {
// Check if the button is pressed
delay(50); // Debounce delay
while (digitalRead(buttonPin) == LOW);
// Wait for button release
inputIndex = 0; /
/ Reset input sequence index
Serial.println("Enter password sequence:");
// Wait for password input
while (inputIndex < passwordLength) {
for (int i = 0; i < 3; i++) {
if (digitalRead(touchPins[i]) == HIGH) {
inputSequence[inputIndex] = i + 1;
// Store the touch sensor number (1, 2, 3)
inputIndex++;
Serial.print("Touch sensor ");
Serial.println(i + 1);
delay(500); // Debounce delay
while (digitalRead(touchPins[i]) == HIGH);
// Wait for sensor release
}
}
}
// Check if the entered sequence matches the password
bool isCorrect = true;
for (int i = 0; i < passwordLength; i++) {
if (inputSequence[i] != password[i]) {
isCorrect = false;
break;
}
}
if (isCorrect) {
digitalWrite(ledPin, HIGH);
// Turn on the LED if password is correct
Serial.println("Password correct!");
} else {
digitalWrite(buzzerPin, HIGH);
// Turn on the buzzer if password is incorrect
Serial.println("Password incorrect!");
delay(5000); // Buzzer on for 5 seconds
digitalWrite(buzzerPin, LOW);
}
delay(5000); // Wait 5 seconds before resetting
digitalWrite(ledPin, LOW);
// Turn off the LED
Serial.println("Ready for new input.");
}
}
Loop Function
- Button Press Detection:
- Continuously checks if the button is pressed (digitalRead(buttonPin) == LOW).
- A debounce delay of 50 milliseconds is used to ensure the button press is registered correctly.
- Waits for the button to be released before continuing.
- Resetting Input Sequence:
- Resets the input index to 0.
- Prints a prompt to the Serial Monitor asking the user to enter the password sequence.
- Reading Touch Sensor Inputs:
- Waits for the user to input the password sequence.
- Checks each touch sensor (digitalRead(touchPins[i]) == HIGH).
- If a touch is detected, the corresponding sensor number (1, 2, or 3) is stored in inputSequence, and the index is incremented.
- A debounce delay of 500 milliseconds is used to avoid multiple readings from a single touch.
- Waits for the touch sensor to be released before continuing.
- Comparing Input Sequence with Password:
- Initializes a boolean variable isCorrect to true.
- Compares each element in inputSequence with the corresponding element in password.
- If any element does not match, isCorrect is set to false, and the loop breaks.
- Providing Feedback:
- If the input sequence matches the password, the LED is turned on (digitalWrite(ledPin, HIGH)) and a message is displayed on the Serial Monitor.
- If the input sequence does not match the password, the buzzer is turned on for 5 seconds (digitalWrite(buzzerPin, HIGH)), then turned off, and a message is displayed on the Serial Monitor.
- Resetting for Next Input:
- Waits for 5 seconds (delay(5000)) before resetting.
- Turns off the LED (digitalWrite(ledPin, LOW)) and prints a message to the Serial Monitor indicating the system is ready for new input.
Try Yourself
Try to add a provision to read the password through the keyboard instead of embedding the password in the program.