Basics of a Joystick
A joystick is an input device commonly used to control video games and robotic systems. It consists of a handle that pivots on two axes (X and Y) and often includes a button switch.
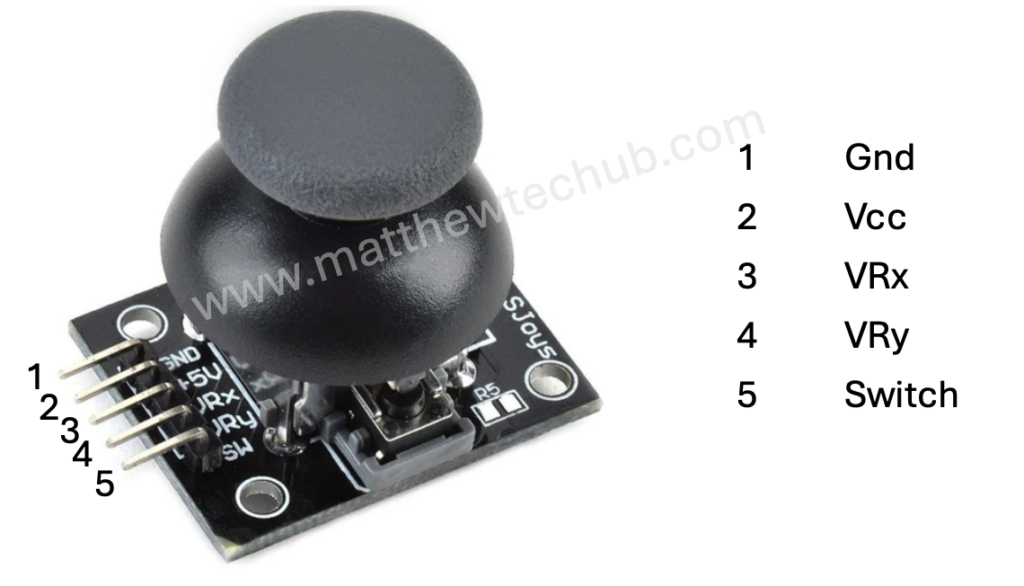
Components of a Joystick
- X-axis and Y-axis Potentiometers:
- These measure the position of the joystick handle along the horizontal (X) and vertical (Y) axes.
- Each potentiometer is essentially a variable resistor whose resistance changes as you move the joystick.
- The changing resistance alters the voltage at the analog output pins of the joystick, which can be read by an analog-to-digital converter (ADC) in a microcontroller.
- Button:
- Many joysticks include a button that can be pressed when the joystick is pushed down.
- This button typically provides a digital output: HIGH when not pressed and LOW when pressed.
Analog Outputs (X and Y axes)
- When the joystick is centered, both potentiometers typically output a voltage that is half of the supply voltage (e.g., 2.5V if powered by 5V).
- Moving the joystick handle changes these voltages. Moving it to one extreme of an axis will give a high voltage (near the supply voltage), and moving it to the other extreme will give a low voltage (near ground).
Circuit Wiring
Program Code
C++
// www.matthewtechub.com
// joystick basics
const int joyXPin = A0; // Analog pin for X-axis
const int joyYPin = A1; // Analog pin for Y-axis
const int joyButtonPin = 2; // Digital pin for the button
void setup() {
Serial.begin(9600); // Initialize serial communication at 9600 bps
pinMode(joyButtonPin, INPUT_PULLUP); // Set button pin as input with internal pull-up
}
void loop() {
int joyXValue = analogRead(joyXPin); // Read X-axis value
int joyYValue = analogRead(joyYPin); // Read Y-axis value
int joyButtonState = digitalRead(joyButtonPin); // Read button state
Serial.print("X-axis: ");
Serial.print(joyXValue);
Serial.print(" | Y-axis: ");
Serial.print(joyYValue);
Serial.print(" | Button: ");
Serial.println(joyButtonState == LOW ? "Pressed" : "Released");
delay(1000); // Small delay before next read
}
By connecting your joystick to the specified pins and running this code on your Arduino, you can monitor the joystick’s movement and button state via the Serial Monitor.
Code Explanation
C++
const int joyXPin = A0; // Analog pin for X-axis
const int joyYPin = A1; // Analog pin for Y-axis
const int joyButtonPin = 2; // Digital pin for the button
Variable Declarations and Pin Definitions
- joyXPin: Analog pin A0 is used to read the X-axis position of the joystick.
- joyYPin: Analog pin A1 is used to read the Y-axis position of the joystick.
- joyButtonPin: Digital pin 2 is used to read the state of the joystick button.
C++
void setup() {
Serial.begin(9600); // Initialize serial communication at 9600 bps
pinMode(joyButtonPin, INPUT_PULLUP); // Set button pin as input with internal pull-up
}
Setup Function
- Serial.begin(9600);: Initializes serial communication at 9600 bits per second. This is used to send data to the Serial Monitor.
- pinMode(joyButtonPin, INPUT_PULLUP);: Sets the button pin as an input with an internal pull-up resistor. This means the pin will read HIGH when the button is not pressed and LOW when it is pressed.
C++
void loop() {
int joyXValue = analogRead(joyXPin); // Read X-axis value
int joyYValue = analogRead(joyYPin); // Read Y-axis value
int joyButtonState = digitalRead(joyButtonPin); // Read button state
Serial.print("X-axis: ");
Serial.print(joyXValue);
Serial.print(" | Y-axis: ");
Serial.print(joyYValue);
Serial.print(" | Button: ");
Serial.println(joyButtonState == LOW ? "Pressed" : "Released");
delay(1000); // Small delay before next read
}
Loop Function
- int joyXValue = analogRead(joyXPin);: Reads the analog value from the X-axis potentiometer and stores it in joyXValue.
- int joyYValue = analogRead(joyYPin);: Reads the analog value from the Y-axis potentiometer and stores it in joyYValue.
- int joyButtonState = digitalRead(joyButtonPin);: Reads the digital state of the joystick button and stores it in joyButtonState. The state will be LOW if the button is pressed and HIGH if it is not pressed.
- Serial.print(): Prints the specified value to the Serial Monitor.
- Serial.println(): Prints the specified value to the Serial Monitor and then moves to a new line.
- The values for X-axis, Y-axis, and button state are printed to the Serial Monitor every second.
delay(1000); // Small delay before next read
Serial Monitor
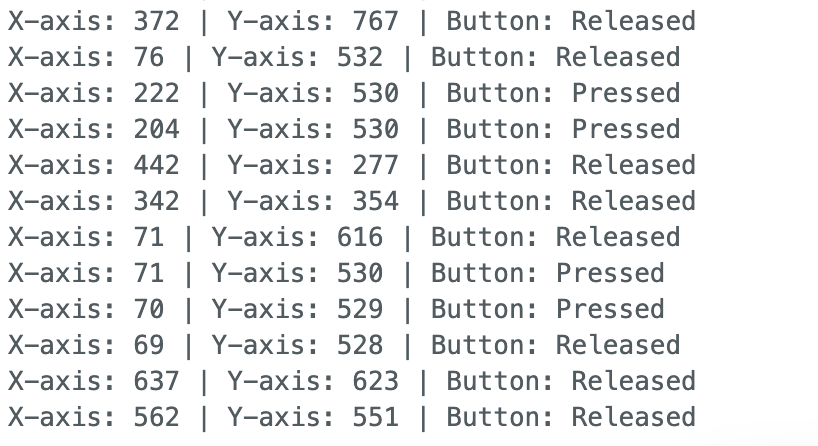