About The Project
In this project, we will learn how to count the number of obstacles detected in front of an IR sensor module and display the count value on the Serial Monitor.
IR Sensor Module
An IR (Infrared) sensor module is a device that detects infrared light to identify objects, measure distances, or detect movement. It typically consists of an IR LED (emitter) and an IR photodiode (receiver) arranged in a way that the IR light emitted by the LED can be reflected back to the receiver when an object is in front of it.
When an object comes within the sensor’s range, the IR light emitted by the LED reflects off the object and is detected by the photodiode. This causes the sensor to change its output, which can then be read by a microcontroller.
Circuit Wiring
Program Code
// www.matthewtechub.com
// IR Sensor- Counter
// Define the digital pin for the IR sensor
const int sensorPin = 2;
// Variables to store sensor state and count
int sensorState = 0;
int lastSensorState = 0;
int objectCount = 0;
void setup() {
// Initialize the digital pin as input
pinMode(sensorPin, INPUT);
// Initialize serial communication
Serial.begin(9600);
}
void loop() {
// Read the current state of the IR sensor
sensorState = digitalRead(sensorPin);
// Check if the sensor detects an object (transition from HIGH to LOW)
if (sensorState == LOW && lastSensorState == HIGH) {
// Increment the object count
objectCount++;
// Print the updated object count
Serial.print("Objects detected: ");
Serial.println(objectCount);
}
// Update the last sensor state
lastSensorState = sensorState;
// Short delay to debounce the sensor reading
delay(50);
}
This Arduino program is designed to count the number of obstacles detected by an IR sensor and display the count on the Serial Monitor. The program monitors the IR sensor’s state and increments a counter each time an obstacle is detected.
Code Explanation
const int sensorPin = 2;
int sensorState = 0;
int lastSensorState = 0;
int objectCount = 0;
- `const int sensorPin = 2;`: This line defines a constant integer variable `sensorPin` and assigns it the value `2`. This indicates that the IR sensor’s output pin is connected to digital pin 2 on the Arduino.
- `int sensorState = 0;`: This variable will hold the current state of the IR sensor. It is initialized to `0` (LOW).
- `int lastSensorState = 0;`: This variable will hold the previous state of the IR sensor. It is used to detect changes in the sensor’s state and is also initialized to `0` (LOW).
- `int objectCount = 0;`: This variable will store the count of detected objects. It is initialized to `0`.
void setup() {
// Initialize the digital pin as input
pinMode(sensorPin, INPUT);
// Initialize serial communication
Serial.begin(9600);
}
- ‘void setup()`: The `setup` function runs once when the Arduino is powered on or reset.
- `pinMode(sensorPin, INPUT);`: This function sets the mode of `sensorPin` (connected to pin 2) as an input. This allows the Arduino to read the state of the IR sensor.
- `Serial.begin(9600);`: This initializes serial communication with a baud rate of `9600` bits per second, enabling the Arduino to send data to the Serial Monitor on your computer.
void loop() {
// Read the current state of the IR sensor
sensorState = digitalRead(sensorPin);
// Check if the sensor detects an object (transition from HIGH to LOW)
if (sensorState == LOW && lastSensorState == HIGH) {
// Increment the object count
objectCount++;
// Print the updated object count
Serial.print("Objects detected: ");
Serial.println(objectCount);
}
// Update the last sensor state
lastSensorState = sensorState;
// Short delay to debounce the sensor reading
delay(50);
}
- `void loop()`: The `loop` function runs continuously after the `setup` function. It contains the main logic of the program.
- `sensorState = digitalRead(sensorPin);`: This line reads the current state of the IR sensor connected to pin 2 using `digitalRead()`. The function returns `HIGH` or `LOW` depending on whether the sensor detects an object (`LOW`) or not (`HIGH`). The result is stored in the `sensorState` variable.
- `if (sensorState == LOW && lastSensorState == HIGH)`: This condition checks if there is a transition from `HIGH` to `LOW`. This indicates that the sensor has just detected an object (since `LOW` means the sensor is blocked by an object). The program only increments the count when such a transition is detected, preventing multiple counts for a single object.
- `objectCount++;`: If the condition is true, this line increments the `objectCount` by `1`, effectively counting the detected object.
- `Serial.print(“Objects detected: “);`: This line sends the text “Objects detected: ” to the Serial Monitor.
- `Serial.println(objectCount);`: This line sends the current value of `objectCount` to the Serial Monitor on a new line, allowing you to see the total number of objects detected so far.
- `lastSensorState = sensorState;`: This line updates `lastSensorState` to the current `sensorState`. This ensures that the program can correctly detect the next transition from `HIGH` to `LOW`.
- `delay(50);`: This introduces a 50-millisecond delay to debounce the sensor reading. Debouncing helps to avoid false counts that could be caused by rapid fluctuations in the sensor’s output.
Screenshot
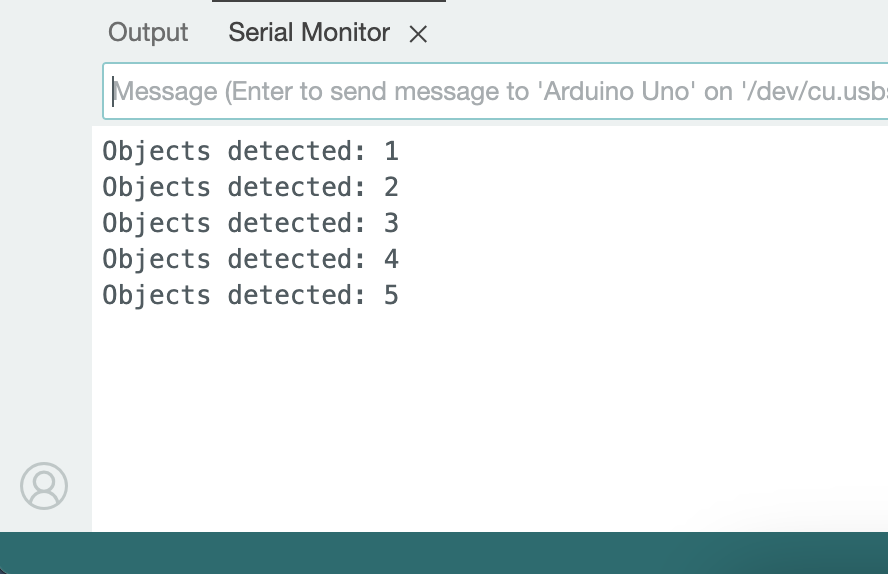