About The Project
In this project, we will learn how to count the number of large objects, such as humans, passing in front of an IR sensor module.
This Arduino program uses two IR sensors to count the number of large objects, such as humans, that pass in front of them simultaneously. The sensors are placed a certain distance apart, and the program only increments the object count when both sensors detect an object at the same time. The count is then displayed on the Serial Monitor.
Why This Approach?
Typically, IR sensor-based Arduino projects count the number of obstacles that pass by, detecting both large objects like humans and small objects like flies or even mosquitoes. However, these systems aren’t effective for counting large objects exclusively, as they also register smaller ones. In this project, we are using a set of IR sensor modules placed a certain distance apart. When a large object, such as a human, passes in front of both sensors, the system detects the simultaneous response. By analysing the output from both sensors, our counting system accurately identifies and counts larger objects like humans, avoiding false counts from smaller obstacles.
IR Sensor Module
An IR (Infrared) sensor module is a device that detects infrared light to identify objects, measure distances, or detect movement. It typically consists of an IR LED (emitter) and an IR photodiode (receiver) arranged in a way that the IR light emitted by the LED can be reflected back to the receiver when an object is in front of it.
When an object comes within the sensor’s range, the IR light emitted by the LED reflects off the object and is detected by the photodiode. This causes the sensor to change its output, which can then be read by a microcontroller.
Circuit Wiring
Program Code
// Define the digital pins for the two IR sensors
const int sensor1Pin = 2;
const int sensor2Pin = 3;
// Variables to store sensor states and the object counter
int sensor1State = 0;
int sensor2State = 0;
int objectCount = 0;
void setup() {
// Initialize the digital pins as inputs
pinMode(sensor1Pin, INPUT);
pinMode(sensor2Pin, INPUT);
// Initialize serial communication
Serial.begin(9600);
}
void loop() {
// Read the current state of both IR sensors
sensor1State = digitalRead(sensor1Pin);
sensor2State = digitalRead(sensor2Pin);
// Check if both sensors detect an object simultaneously
if (sensor1State == LOW && sensor2State == LOW) {
// Increment the object count
objectCount++;
// Print the updated object count
Serial.print("Objects detected: ");
Serial.println(objectCount);
// Wait until the object passes completely before continuing
while(digitalRead(sensor1Pin) == LOW || digitalRead(sensor2Pin) == LOW) {
// Do nothing, just wait
}
}
// Short delay to debounce the sensor readings
delay(50);
}
This program counts the number of large objects (like humans) passing simultaneously in front of two IR sensors placed a certain distance apart. The system increments the count only when both sensors detect the object at the same time, reducing the likelihood of counting small objects or noise. The count is displayed on the Serial Monitor, and the system waits until the object passes completely before checking for the next one.
Code Explanation
// Define the digital pins for the two IR sensors
const int sensor1Pin = 2;
const int sensor2Pin = 3;
- These lines define constant integer variables for the pins connected to the two IR sensors. The first IR sensor is connected to digital pin 2, and the second IR sensor is connected to digital pin 3 on the Arduino.
// Variables to store sensor states and the object counter
int sensor1State = 0;
int sensor2State = 0;
int objectCount = 0;
- `int sensor1State = 0;` and `int sensor2State = 0;`: These variables store the current state of the two IR sensors. They are initialized to `0` (LOW).
- `int objectCount = 0;`: This variable keeps track of the total number of objects detected by both sensors simultaneously. It is initialized to `0`.
void setup() {
// Initialize the digital pins as inputs
pinMode(sensor1Pin, INPUT);
pinMode(sensor2Pin, INPUT);
// Initialize serial communication
Serial.begin(9600);
}
- `void setup()`: The `setup` function runs once when the Arduino is powered on or reset.
- `pinMode(sensor1Pin, INPUT);` and `pinMode(sensor2Pin, INPUT);`: These lines configure the pins connected to the IR sensors as inputs, allowing the Arduino to read the state of each sensor.
- `Serial.begin(9600);`: This initializes serial communication at a baud rate of `9600` bits per second, enabling the Arduino to send data to the Serial Monitor.
void loop() {
// Read the current state of both IR sensors
sensor1State = digitalRead(sensor1Pin);
sensor2State = digitalRead(sensor2Pin);
- `void loop()`: The `loop` function runs continuously after `setup` and contains the main logic of the program.
- `sensor1State = digitalRead(sensor1Pin);` and `sensor2State = digitalRead(sensor2Pin);`: These lines read the current state of the two IR sensors. The `digitalRead()` function returns `HIGH` if no object is detected and `LOW` if an object is detected in front of the sensor. The results are stored in `sensor1State` and `sensor2State`.
// Check if both sensors detect an object simultaneously
if (sensor1State == LOW && sensor2State == LOW) {
// Increment the object count
objectCount++;
// Print the updated object count
Serial.print("Objects detected: ");
Serial.println(objectCount);
- `if (sensor1State == LOW && sensor2State == LOW)`: This condition checks whether both sensors detect an object at the same time. Both sensor states must be `LOW` (meaning both sensors are blocked) for the condition to be true.
- `objectCount++;`: If the condition is true, this line increments the `objectCount` by `1`, reflecting the detection of a large object.
- `Serial.print(“Objects detected: “);` and `Serial.println(objectCount);`: These lines send the text “Objects detected: ” followed by the current value of `objectCount` to the Serial Monitor, displaying the total number of detected objects.
// Wait until the object passes completely before continuing
while(digitalRead(sensor1Pin) == LOW || digitalRead(sensor2Pin) == LOW) {
// Do nothing, just wait
}
}
- `while(digitalRead(sensor1Pin) == LOW || digitalRead(sensor2Pin) == LOW)`: This `while` loop keeps the program in a waiting state until the object passes completely (i.e., neither sensor detects the object anymore). It continues to check the state of both sensors and exits the loop only when both sensors return to `HIGH` (unblocked).
- `// Do nothing, just wait`: During this waiting period, the program does nothing except keep checking the sensor states. This ensures that the system doesn’t count the same object multiple times as it moves past the sensors.
// Short delay to debounce the sensor readings
delay(50);
}
- ‘delay(50);`: This introduces a short delay of 50 milliseconds to debounce the sensor readings. Debouncing helps to filter out any noise or fluctuations in the sensor signals, ensuring more accurate counting.
Screenshot
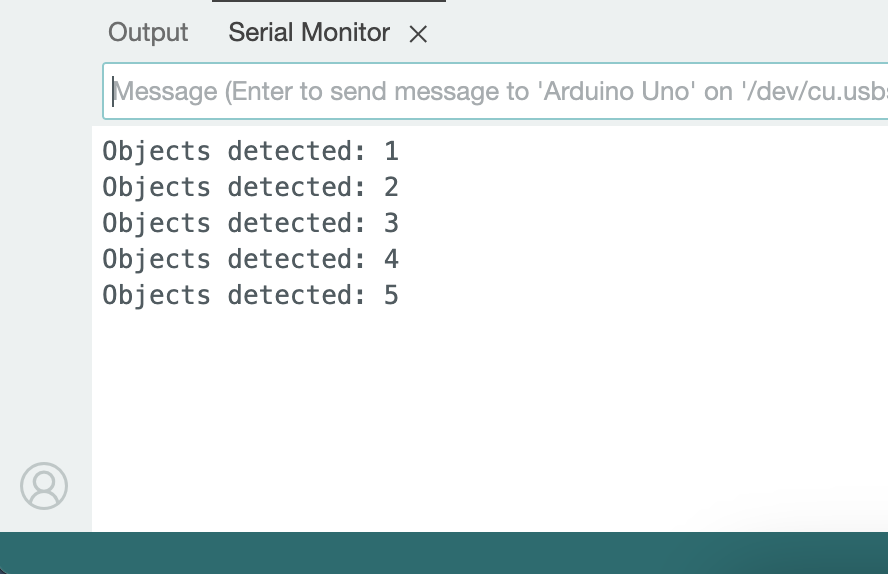