About The Project
This project is a burglar alarm system designed to operate only during nighttime or in low-light conditions. It uses a light sensor to detect darkness and a PIR sensor to identify motion, triggering a buzzer if unauthorized movement is detected.
Scope of The Project
The scope of this project includes enhancing home security by automating an alarm system that only activates during night or low-light conditions. It can be implemented in residential or commercial spaces to detect and deter unauthorized entry after dark.
PIR Sensor
A PIR (Passive Infrared) sensor is a type of motion sensor that detects infrared (IR) radiation emitted by objects within its field of view, particularly living beings like humans and animals.
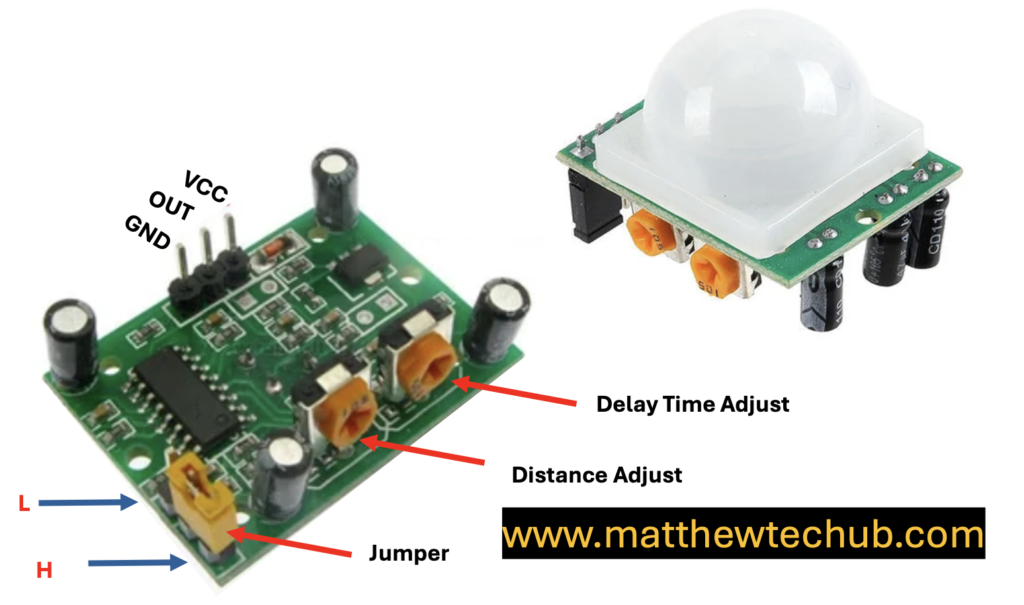
How PIR Sensor Output Works:
- Motion Detected (Moving Into or Within the Range):
– Output: The sensor’s output goes HIGH when it detects motion, regardless of whether the motion is towards or away from the sensor. This HIGH state indicates that some movement has been detected within the sensor’s field of view.
- Continued Motion:
– Output: If the object continues to move within the sensor’s detection range, the output will remain HIGH as long as the motion is detected.
- Motion Stops or Object Leaves the Range:
– Output: Once the motion stops or the object moves out of the sensor’s range, the sensor’s output will stay HIGH for a brief period (based on the sensor’s time delay setting) and then return to LOW. The transition from HIGH to LOW does not occur specifically because the object moved away; it happens because the motion has stopped being detected.
LDR
A Light Dependent Resistor (LDR), also known as a photoresistor or photoconductor, is a type of resistor whose resistance varies significantly with the amount of light falling on its surface.
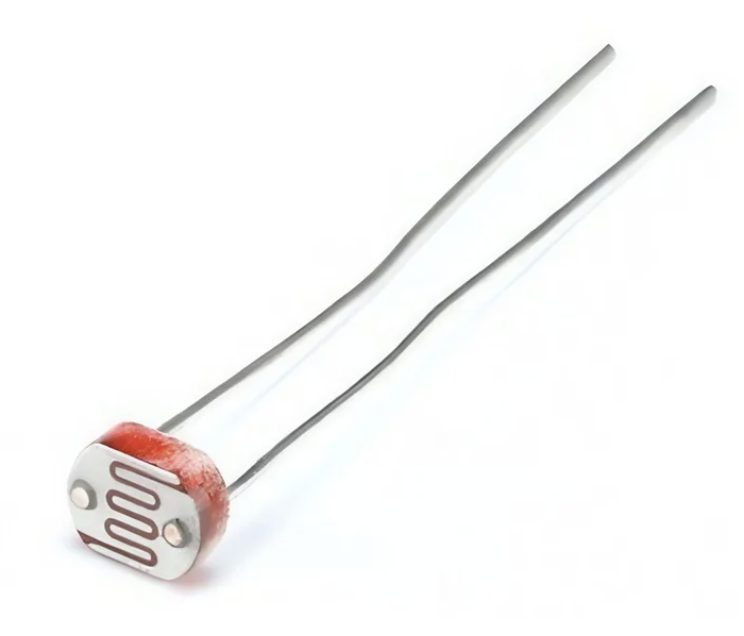
Voltage Dividing Circuit
A voltage divider circuit is used in light measurement with an LDR (Light Dependent Resistor) to convert the varying resistance of the LDR into a measurable voltage. The Arduino can read voltage levels through its analog input pins but cannot directly measure resistance.
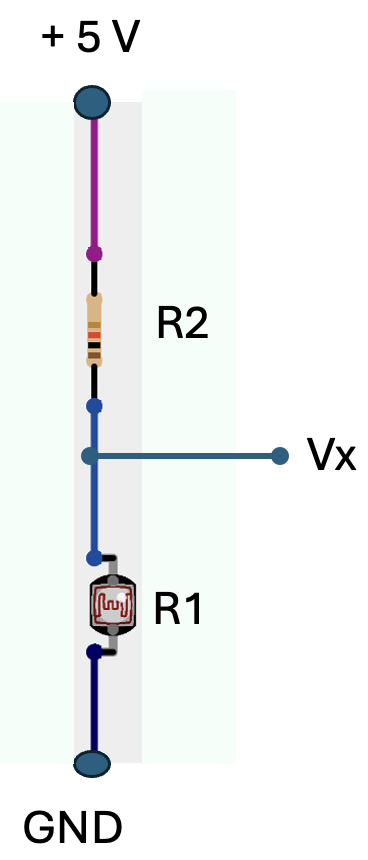
The Arduino reads this voltage (Vx) as an analog input, converting it to a digital value using its Analog to Digital Converter (ADC). The ADC outputs a value between 0 and 1023, corresponding to a voltage range of 0 to 5V.
Circuit Wiring
The circuit is designed to create a burglar alarm system that only operates in darkness. It uses a light sensor (LDR) to detect the ambient light level and a PIR (Passive Infrared) sensor to detect motion. If the light level is high (indicating night time) and motion is detected, the system triggers a buzzer to sound the alarm.
Program Code
// www.matthewtechub.com
// Burglar Alarm System for Night Time with Light Sensor and PIR Sensor
const int lightSensorPin = A0; // Analog pin for light sensor (LDR)
const int pirPin = 2; // Digital pin for PIR sensor
const int buzzerPin = 3; // Digital pin for buzzer
const int lightThreshold = 950; // Light level below which the system is active (darkness threshold)
int lightLevel; // Variable to store the light sensor value
int pirState; // Variable to store the PIR sensor state
void setup() {
Serial.begin(9600); // Initialize serial communication at 9600 bps
pinMode(pirPin, INPUT); // Set the PIR sensor pin as input
pinMode(buzzerPin, OUTPUT); // Set the buzzer pin as output
}
void loop() {
lightLevel = analogRead(lightSensorPin); // Read the light level from the light sensor
// Print light level for debugging
Serial.print("Light Level: ");
Serial.println(lightLevel);
// Check if it is dark
if (lightLevel > lightThreshold) {
// It is dark, check for motion
pirState = digitalRead(pirPin); // Read the state of the PIR sensor
// If motion is detected
if (pirState == HIGH) {
Serial.println("Motion detected in darkness!"); // Print message to Serial Monitor
digitalWrite(buzzerPin, HIGH); // Turn on the buzzer
} else {
Serial.println("No motion detected."); // Print message to Serial Monitor when no motion
digitalWrite(buzzerPin, LOW); // Turn off the buzzer
}
} else {
// It is not dark, turn off the buzzer and print status
Serial.println("Light detected, system inactive."); // Print message to Serial Monitor
digitalWrite(buzzerPin, LOW); // Ensure the buzzer is off
}
delay(500); // Delay for stability
}
This code effectively creates a burglar alarm system that only triggers when it’s dark and motion is detected. The Serial Monitor outputs the current light level, motion detection status, and whether the system is active, making it easy to monitor the system’s operation during testing or deployment.
Code Explanation
const int lightSensorPin = A0; // Analog pin for light sensor (LDR)
const int pirPin = 2; // Digital pin for PIR sensor
const int buzzerPin = 3; // Digital pin for buzzer
const int lightThreshold = 950; // Light level below which the system is active (darkness threshold)
int lightLevel; // Variable to store the light sensor value
int pirState; // Variable to store the PIR sensor state
- `lightSensorPin`: The analog pin A0 is connected to the LDR (Light Dependent Resistor), which measures the ambient light level.
- `pirPin`: Digital pin 2 is connected to the PIR sensor, which detects motion.
- `buzzerPin`: Digital pin 3 is connected to a buzzer, which will sound when motion is detected.
- `lightThreshold`: A threshold value (950) that determines when it is considered “dark” enough for the system to be active.
- `lightLevel`: Stores the current light level read from the LDR.
- `pirState`: Stores the current state of the PIR sensor (HIGH if motion is detected, LOW if no motion is detected).
void setup() {
Serial.begin(9600); // Initialize serial communication at 9600 bps
pinMode(pirPin, INPUT); // Set the PIR sensor pin as input
pinMode(buzzerPin, OUTPUT); // Set the buzzer pin as output
}
- `Serial.begin(9600);`: Initializes the serial communication at a baud rate of 9600, allowing data to be printed to the Serial Monitor.
- `pinMode(pirPin, INPUT);`: Sets the PIR sensor pin as an input, allowing the Arduino to read whether motion is detected.
- `pinMode(buzzerPin, OUTPUT);`: Sets the buzzer pin as an output, allowing the Arduino to control the buzzer.
void loop() {
lightLevel = analogRead(lightSensorPin); // Read the light level from the light sensor
// Print light level for debugging
Serial.print("Light Level: ");
Serial.println(lightLevel);
- `lightLevel = analogRead(lightSensorPin);`: Reads the current light level from the LDR and stores it in `lightLevel`.
- `Serial.print` and `Serial.println`: Print the current light level to the Serial Monitor for debugging purposes.
if (lightLevel > lightThreshold) {
// It is dark, check for motion
pirState = digitalRead(pirPin); // Read the state of the PIR sensor
- `if (lightLevel > lightThreshold)`: Checks if the current light level is above the threshold, indicating darkness.
- `pirState = digitalRead(pirPin);`: Reads the current state of the PIR sensor. If it detects motion, `pirState` will be HIGH.
// If motion is detected
if (pirState == HIGH) {
Serial.println("Motion detected in darkness!"); // Print message to Serial Monitor
digitalWrite(buzzerPin, HIGH); // Turn on the buzzer
} else {
Serial.println("No motion detected."); // Print message to Serial Monitor when no motion
digitalWrite(buzzerPin, LOW); // Turn off the buzzer
}
} else {
// It is not dark, turn off the buzzer and print status
Serial.println("Light detected, system inactive."); // Print message to Serial Monitor
digitalWrite(buzzerPin, LOW); // Ensure the buzzer is off
}
delay(500); // Delay for stability
}
- `if (pirState == HIGH)`: If motion is detected, it prints “Motion detected in darkness!” to the Serial Monitor and turns on the buzzer by setting `buzzerPin` to HIGH.
- `else`: If no motion is detected, it prints “No motion detected.” to the Serial Monitor and turns off the buzzer by setting `buzzerPin` to LOW.
- `else`: If the light level is not below the threshold (meaning it’s not dark enough), the system ensures the buzzer is off and prints “Light detected, system inactive.” to the Serial Monitor.
- `delay(500);`: Adds a 500-millisecond delay to prevent the loop from running too rapidly, ensuring stable operation.
Screenshot
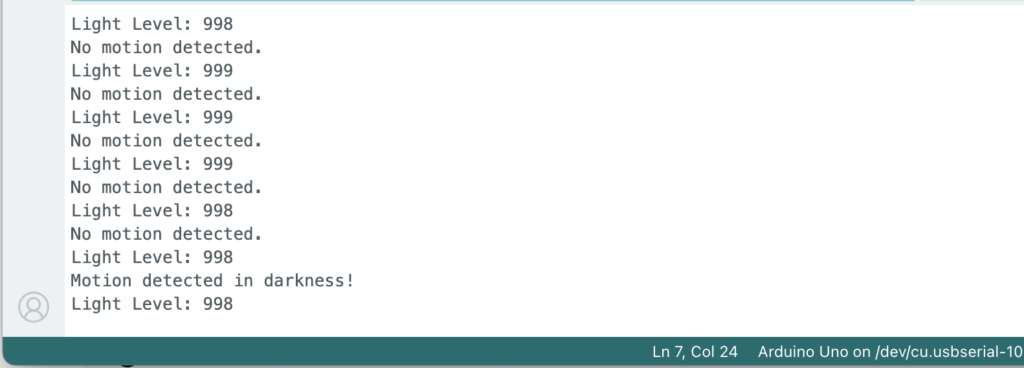