About The Project
This project demonstrates how to interface a PIR motion sensor with an Arduino to detect movement and trigger actions. When motion is detected, an LED is turned on, and when motion stops, the LED is turned off, providing a visual indication of activity.
Scope of The Project
The scope of this project includes creating a basic motion detection system that can be used in security applications, such as automatically turning on lights when movement is detected. Additionally, it serves as a foundation for more advanced automation projects, including integrating alarms or triggering cameras based on motion.
PIR Sensor
A PIR (Passive Infrared) sensor is a type of motion sensor that detects infrared (IR) radiation emitted by objects within its field of view, particularly living beings like humans and animals.
How PIR Sensor Output Works:
- Motion Detected (Moving Into or Within the Range):
– Output: The sensor’s output goes HIGH when it detects motion, regardless of whether the motion is towards or away from the sensor. This HIGH state indicates that some movement has been detected within the sensor’s field of view.
- Continued Motion:
– Output: If the object continues to move within the sensor’s detection range, the output will remain HIGH as long as the motion is detected.
- Motion Stops or Object Leaves the Range:
– Output: Once the motion stops or the object moves out of the sensor’s range, the sensor’s output will stay HIGH for a brief period (based on the sensor’s time delay setting) and then return to LOW. The transition from HIGH to LOW does not occur specifically because the object moved away; it happens because the motion has stopped being detected.
Circuit Wiring
Program Code
This program is designed to interface a PIR (Passive Infrared) motion sensor with an Arduino. When motion is detected, it turns on an LED and sends a message to the Serial Monitor. When motion stops, the LED is turned off, and another message is sent to the Serial Monitor.
// www.matthewtechub.com
// PIR motion detection with LED indication
const int pirPin = 2; // PIR sensor output pin connected to digital pin 2
const int ledPin = 13; // LED connected to digital pin 13 (built-in LED on most Arduino boards)
int pinStateCurrent = LOW; // Current state of the PIR sensor output
int pinStatePrevious = LOW; // Previous state of the PIR sensor output
void setup() {
Serial.begin(9600); // Initialize serial communication at 9600 bps
pinMode(pirPin, INPUT); // Set the PIR sensor pin as an input
pinMode(ledPin, OUTPUT); // Set the LED pin as an output
}
void loop() {
pinStatePrevious = pinStateCurrent; // Save the previous state of the PIR sensor
pinStateCurrent = digitalRead(pirPin); // Read the current state of the PIR sensor
// If motion is detected (LOW -> HIGH transition)
if (pinStatePrevious == LOW && pinStateCurrent == HIGH) {
Serial.println("Motion detected!"); // Print message to Serial Monitor
digitalWrite(ledPin, HIGH); // Turn on the LED
}
// If motion stops (HIGH -> LOW transition)
else if (pinStatePrevious == HIGH && pinStateCurrent == LOW) {
Serial.println("Motion stopped!"); // Print message to Serial Monitor
digitalWrite(ledPin, LOW); // Turn off the LED
}
}
Code Explanation
const int pirPin = 2; // PIR sensor output pin connected to digital pin 2
const int ledPin = 13; // LED connected to digital pin 13 (built-in LED on most Arduino boards)
int pinStateCurrent = LOW; // Current state of the PIR sensor output
int pinStatePrevious = LOW; // Previous state of the PIR sensor output
- `pirPin`: This constant stores the pin number (digital pin 2) where the output pin of the PIR sensor is connected.
- `ledPin`: This constant stores the pin number (digital pin 13) where the LED is connected. Most Arduino boards have a built-in LED connected to pin 13.
- `pinStateCurrent`: A variable that stores the current state of the PIR sensor’s output (either `HIGH` or `LOW`).
- `pinStatePrevious`: A variable that stores the previous state of the PIR sensor’s output, which helps in detecting changes in the state.
void setup() {
Serial.begin(9600); // Initialize serial communication at 9600 bps
pinMode(pirPin, INPUT); // Set the PIR sensor pin as an input
pinMode(ledPin, OUTPUT); // Set the LED pin as an output
}
- `Serial.begin(9600);`: Initializes serial communication at a baud rate of 9600 bits per second, allowing the Arduino to send data to the Serial Monitor.
- `pinMode(pirPin, INPUT);`: Configures the `pirPin` (connected to the PIR sensor) as an input so the Arduino can read the sensor’s state.
- `pinMode(ledPin, OUTPUT);`: Configures the `ledPin` (connected to the LED) as an output so the Arduino can control the LED.
void loop() {
pinStatePrevious = pinStateCurrent; // Save the previous state of the PIR sensor
pinStateCurrent = digitalRead(pirPin); // Read the current state of the PIR sensor
- `pinStatePrevious = pinStateCurrent;`: Before updating the current state of the sensor, the code saves the previous state. This is important for detecting changes in the sensor’s output.
- `pinStateCurrent = digitalRead(pirPin);`: Reads the current state of the PIR sensor (either `HIGH` or `LOW`) and stores it in `pinStateCurrent`.
Motion Detection Logic
// If motion is detected (LOW -> HIGH transition)
if (pinStatePrevious == LOW && pinStateCurrent == HIGH) {
Serial.println("Motion detected!"); // Print message to Serial Monitor
digitalWrite(ledPin, HIGH); // Turn on the LED
}
- `if (pinStatePrevious == LOW && pinStateCurrent == HIGH)`: This condition checks if the PIR sensor’s state has changed from `LOW` (no motion) to `HIGH` (motion detected). This indicates that motion has just been detected.
- `Serial.println(“Motion detected!”);`: Sends the message “Motion detected!” to the Serial Monitor, which allows the user to see the output.
- `digitalWrite(ledPin, HIGH);`: Turns on the LED by setting the `ledPin` to `HIGH`.
Motion Stopped Logic
// If motion stops (HIGH -> LOW transition)
else if (pinStatePrevious == HIGH && pinStateCurrent == LOW) {
Serial.println("Motion stopped!"); // Print message to Serial Monitor
digitalWrite(ledPin, LOW); // Turn off the LED
}
}
- `else if (pinStatePrevious == HIGH && pinStateCurrent == LOW)`: This condition checks if the PIR sensor’s state has changed from `HIGH` (motion detected) to `LOW` (no motion). This indicates that motion has just stopped.
- `Serial.println(“Motion stopped!”);`: Sends the message “Motion stopped!” to the Serial Monitor, informing the user that the motion has ceased.
- `digitalWrite(ledPin, LOW);`: Turns off the LED by setting the `ledPin` to `LOW`.
Screenshot
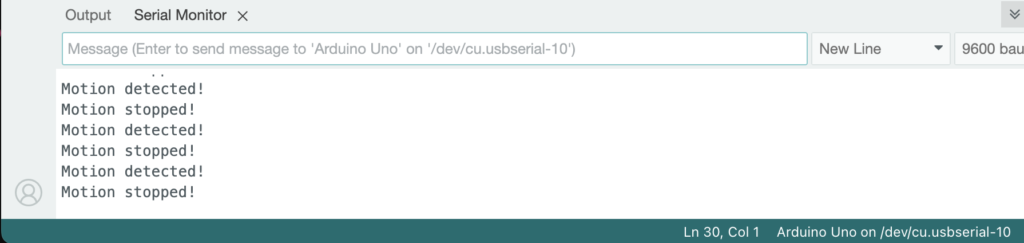